Policy Learner by Athey and Wager (2018) with Binary Treatment
This notebook demonstrates the use of the CausalML implementation of the policy learner by Athey and Wager (2018) (https://arxiv.org/abs/1702.02896).
[1]:
%load_ext autoreload
%autoreload 2
[2]:
import pandas as pd
import numpy as np
from matplotlib import pyplot as plt
[3]:
from sklearn.model_selection import cross_val_predict, KFold
from sklearn.ensemble import GradientBoostingRegressor, GradientBoostingClassifier
from sklearn.tree import DecisionTreeClassifier
[4]:
from causalml.optimize import PolicyLearner
from sklearn.tree import plot_tree
from lightgbm import LGBMRegressor
from causalml.inference.meta import BaseXRegressor
---------------------------------------------------------------------------
RuntimeError Traceback (most recent call last)
RuntimeError: module compiled against API version 0xe but this version of numpy is 0xd
The sklearn.utils.testing module is deprecated in version 0.22 and will be removed in version 0.24. The corresponding classes / functions should instead be imported from sklearn.utils. Anything that cannot be imported from sklearn.utils is now part of the private API.
Binary treatment policy learning
First we generate a synthetic data set with binary treatment. The treatment is random conditioned on covariates. The treatment effect is heterogeneous where for some individuals it is negative. We use a policy learner to classify the individuals into treat/no-treat groups to maximize the total treatment effect.
[5]:
np.random.seed(1234)
n = 10000
p = 10
X = np.random.normal(size=(n, p))
ee = 1 / (1 + np.exp(X[:, 2]))
tt = 1 / (1 + np.exp(X[:, 0] + X[:, 1])/2) - 0.5
W = np.random.binomial(1, ee, n)
Y = X[:, 2] + W * tt + np.random.normal(size=n)
Use policy learner with default outcome/treatment estimator and a simple policy classifier.
[6]:
policy_learner = PolicyLearner(policy_learner=DecisionTreeClassifier(max_depth=2), calibration=True)
[7]:
policy_learner.fit(X, W, Y)
[7]:
PolicyLearner(model_mu=GradientBoostingRegressor(),
model_w=GradientBoostingClassifier(),
\model_pi=DecisionTreeClassifier(max_depth=2))
[8]:
plt.figure(figsize=(15,7))
plot_tree(policy_learner.model_pi)
[8]:
[Text(469.8, 340.2, 'X[0] <= -0.938\ngini = 0.497\nsamples = 10000\nvalue = [7453.281, 8811.792]'),
Text(234.9, 204.12, 'X[5] <= -0.396\ngini = 0.461\nsamples = 1760\nvalue = [1090.326, 1941.877]'),
Text(117.45, 68.03999999999996, 'gini = 0.489\nsamples = 619\nvalue = [428.371, 575.3]'),
Text(352.35, 68.03999999999996, 'gini = 0.44\nsamples = 1141\nvalue = [661.956, 1366.577]'),
Text(704.7, 204.12, 'X[1] <= 0.035\ngini = 0.499\nsamples = 8240\nvalue = [6362.955, 6869.915]'),
Text(587.25, 68.03999999999996, 'gini = 0.491\nsamples = 4252\nvalue = [2957.726, 3857.306]'),
Text(822.15, 68.03999999999996, 'gini = 0.498\nsamples = 3988\nvalue = [3405.228, 3012.609]')]
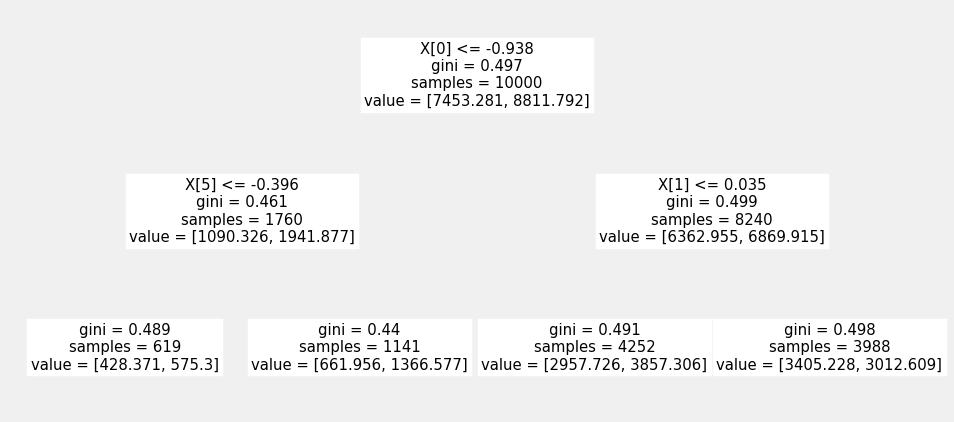
Alternatively, one can construct a policy directly from the ITE estimated from a X-learner.
[9]:
learner_x = BaseXRegressor(LGBMRegressor())
ite_x = learner_x.fit_predict(X=X, treatment=W, y=Y)
In this example policy learner outperforms the ITE-based policy and gets close to the true optimal.
[10]:
pd.DataFrame({
'DR-DT Optimal': [np.mean((policy_learner.predict(X) + 1) * tt / 2)],
'True Optimal': [np.mean((np.sign(tt) + 1) * tt / 2)],
'X Learner': [
np.mean((np.sign(ite_x) + 1) * tt / 2)
],
})
[10]:
DR-DT Optimal | True Optimal | X Learner | |
---|---|---|---|
0 | 0.157055 | 0.183291 | 0.083172 |
[ ]: